I needed an easy way to make Wifi connection on my Raspberry Pi, without the need to remember lines of 40+ chars with parameters, that you don't even know or remember while typing. Also it had to be on the terminal and because nmtui
didn't fit in the little screen of my cyberdeck, i had to invent another way.
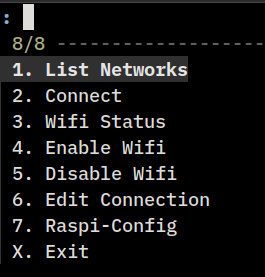
The script uses nmcli
and fzf
and can run on any sized terminals. You can list available networks, select them and make a connection, enable/disable the wifi adapter and edit an existing connection. Just for convenience you can also run raspi-config
.
Edit 2024/10/29:
Added some more features.
- Show saved connections
- Connect to saved and new connections
- Show Wifi status
#!/bin/bash
#title : fzf script for wifi connection on Raspberry Pi
#description :
#author : xqtr
#date : 2024/10/24
#version : 1.0
#usage :
#notes : needs nmcli and fzf
function presskey() {
echo ""
echo "Press a key to continue..."
read -n 1
}
function connect_new() {
clear
wlan=$(nmcli dev wifi list | awk '{print $2, $3, $6, $7, $9, $10}' | fzf --header "Select to connect. ESC to exit.")
if [ -z "$wlan" ]; then
echo "Exiting... "
return -1
fi
clear
echo "$wlan"
echo "--------------------"
sudo nmcli --ask dev wifi connect "$(echo $wlan | cut -d" " -f 1)"
presskey
}
function connect_saved() {
clear
wlan=$(sudo nmcli c show | awk '{print $1, $3, $4}' | fzf --header "Select to connect. ESC to exit.")
if [ -z "$wlan" ]; then
echo "Exiting... "
return -1
fi
clear
echo "$wlan"
echo "--------------------"
sudo nmcli dev wifi connect "$(echo $wlan | cut -d" " -f 1)"
presskey
}
function wifi_list() {
clear
nmcli dev wifi list | awk '{print $2, $3, $6, $7, $9, $10}' | fzf
}
function wifi_status() {
clear
nmcli dev status
presskey
}
function wifi_enabled() {
clear
nmcli radio wifi
presskey
}
function edit_connection() {
clear
con=$(ls /etc/NetworkManager/system-connections/ | fzf --header "Select to edit...")
if [ ! -z "$con" ]; then
sudo nano /etc/NetworkManager/system-connections/$con
fi
}
function wifi_close(){
clear
con=$(nmcli dev status | fzf --header "Select Connection")
if [ ! -z "$con" ]; then
echo "Closing connection: $(echo "$con" | awk -F ' ' '{print $4}')"
sudo nmcli con down id $(echo "$con" | awk -F ' ' '{print $4}')
fi
presskey
}
option=""
while [ -z "$option" ]
do
option=$(echo "1. List Networks
2. List Avail. Networds and Connect
3. Wifi Status
4. Enable Wifi
5. Disable Wifi
6. Edit Connection
7. Show Connections
8. Is Wifi Enabled
9. Close Connection
0. Enable saved connection
R. Raspi-Config
X. Exit" | fzf)
if [ ! -z "$option" ]
then
ch="$option"
case $ch in
1.*) wifi_list;;
2.*) connect_new;;
3.*) wifi_status;;
4.*) clear;nmcli radio wifi on;wifi_status;;
5.*) clear;nmcli radio wifi off;wifi_status;;
6.*) edit_connection;;
7.*) sudo nmcli c show | fzf;;
8.*) wifi_enabled;;
9.*) wifi_close;;
0.*) connect_saved;;
R.*) sudo raspi-config;;
X.*) exit;;
esac
# Cancel is pressed
#else
# exec $maindir/main.sh
fi
option=""
done